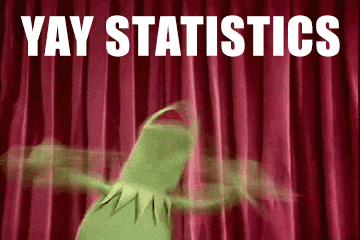
Why do so many machine learning practitioners jump straight into deep learning without understanding the fundamentals of statistical learning? Let me share my experience as a computer science student who ventured into a mathematics-heavy statistical learning course at NTNU.
The Foundation of Machine Learning
Statistical learning forms the theoretical backbone of modern machine learning. While neural networks might seem more exciting, understanding concepts like maximum likelihood estimation and hypothesis testing provides crucial insights into how learning algorithms actually work.
Statistical Learning Theory
At its core, statistical learning deals with inferring predictive functions from data. Consider a probability space $(X \times Y, P)$ where $X$ represents our input space and $Y$ our output space. Here, $P$ is a probability measure that describes how our data is distributed over the input-output space. Our goal is to find a function $f : X \to Y$ that minimizes the expected risk:
$$R(f) = \int_{X \times Y} L(f(x),y) , dP(x,y)$$
where $L$ is our loss function. This framework unifies seemingly distinct learning problems, from simple linear regression to complex neural networks.
The Role of Statistics in Learning
Statistical learning isn’t just about theoretical frameworks - it provides practical tools for:
Model Validation
- Hypothesis testing for feature significance
- Confidence intervals for predictions
- Cross-validation for model selection
Distribution Analysis
- Understanding data generating processes
- Detecting outliers and anomalies
- Quantifying uncertainty in predictions
A Practical Example
Let’s implement a simple yet powerful statistical learning method - linear regression with statistical analysis:
1 | import numpy as np |
This implementation goes beyond simple prediction by incorporating statistical inference. The standard errors and $p$-values help us understand the reliability of our model’s coefficients.
Why Statistics Matter
Statistical learning provides three key advantages:
- Interpretability: Statistical methods give us tools to understand what our models are actually learning.
- Validation: Statistical tests help verify whether our models are capturing meaningful patterns rather than noise.
- Efficiency: Many statistical learning algorithms are computationally simpler yet equally effective for certain tasks compared to deep learning approaches.
Mathematical Foundations
The variance-bias decomposition of prediction error is a fundamental concept in statistical learning. Let’s break down what each term means:
- $Y$ represents the true target value we’re trying to predict
- $\hat{f}$ is our model’s estimator function
- $X$ represents our input features
- $E[(Y - \hat{f}(X))^2]$ is the expected squared prediction error
- $\text{Var}(\hat{f}(X))$ represents how much our predictions vary across different training sets
- $\text{Bias}(\hat{f}(X))$ measures how far off our predictions are from the true function on average
- $\sigma^2$ is the irreducible error due to noise in the data
We can write this decomposition as:
$$E[(Y - \hat{f}(X))^2] = \text{Var}(\hat{f}(X)) + [\text{Bias}(\hat{f}(X))]^2 + \sigma^2$$
This fundamental decomposition helps us understand the trade-off between model complexity and generalization.
From Theory to Practice
Let’s examine how statistical concepts translate into practical machine learning. Here’s an example of implementing cross-validation with statistical significance testing:
1 | from sklearn.model_selection import KFold |
This implementation combines modern machine learning practices with statistical rigor, providing not just performance metrics but also confidence intervals for our results.
Statistical learning isn’t just a prerequisite for machine learning - it’s an essential toolkit that helps us build more reliable and interpretable models. The next time you’re tempted to skip the mathematical foundations and jump straight into deep learning, remember that understanding statistical learning principles might be the key to building better models.